Webhooks Overview
VoPay provides the option to create HTTP callbacks via a POST request, commonly called webhooks or push notifications. These events are triggered when some changes occur in your application at any time. For example, if your transaction has been approved or changes status, we will notify you about that change.
Currently, our webhooks are linked only to changes in the transaction status (not creation or scheduled transactions). This functionality will be expanded in future versions.
How it works
To receive the notification, you have to set up the URL properly. After this configuration occurs, the system will start to send notifications.
The system will attempt to send the webhook three times, expecting a 200 HTTP status code to mark the webhook as a success. If in the first attempt the webhook takes longer than 5 seconds, or it fails, the system will wait five minutes to do the second attempt but this time, it will wait for 15 seconds to get a successful response. If it fails again, it will try 5 minutes later and wait 30 seconds to get a successful response. After the third attempt, the webhook will be considered as deprecated and it will not try again.
The request
To have a clear idea of what you will receive from us, this is an expected response request when a new event will occur in your application. Remember that all our responses are in JSON format.
{
"Success": "true",
"TransactionType": "EFT Funding",
"TransactionID": "1",
"TransactionAmount": "1",
"Status": "in progress",
"ScheduledTransactionID": "1"
"UpdatedAt": "2020-01-01 00:00:00",
"ValidationKey": "0eab3450c5f5509212612ecef3056fe4f0505783"
"FailureReason": "",
"Environment": "[Production, Sandbox]"
}
Set up your webhook
To set up your webhook. You need to use our endpoint POST account/webhook-url send the authentication detail and your webhook URL and you webhook will be ready to use.
$ch = curl_init();
curl_setopt($ch, CURLOPT_URL, "https://earthnode-dev.vopay.com/api/v2/account/webhook-url");
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, array('Content-Type:application/json'));
curl_setopt($ch, CURLOPT_POSTFIELDS, array(
"AccountID" => "myaccount",
"Key" => "aae103d7a897358a65afeee30720f000f6d8eb6a",
"Signature" => "aae103d7a897358a65afeee30720f000f6d8eb6a",
"WebHookUrl" => "http://www.mysite.com/webhook-listener"
));
curl_exec($ch);
The Validation Key
To confirm that the data that we are sending is authentic and comes from VoPay rather than a party pretending to be VoPay we will send you a ValidationKey. This ValidationKey is a combination of your API shared secret key and the transaction ID codified by HMAC SHA1
function calculateKey($apiSharedsecret, $transactionID)
{
return sha1($apiSharedsecret . $transactionID);
}
$calculateKey = calculateKey('IUAz1NGoyM02VsoODfIzQA==', '1');
if ($calculateKey == $validationKey) {
// Validation Key Ok.
} else {
// Invalid Validation Key. Ignore the webhook
}
Expected Transaction Statuses
The status notifications available are as follows:
- pending: The transaction has been created and waiting to send it to the bank.
- in progress: The transaction has been sent to the bank and waiting for the bank response.
- failed: The transaction is failed for wrong information, rejected from the bank, non-sufficient funds, etc.
- cancelled: The transaction is marked as a cancel from the user
- successful: The transaction has been completed and the funds have been released.
These statuses may vary during the lifecycle of the transaction, passing from pending to successful, cancel, or failed. As an overview, this is the general workflow of the transactions.
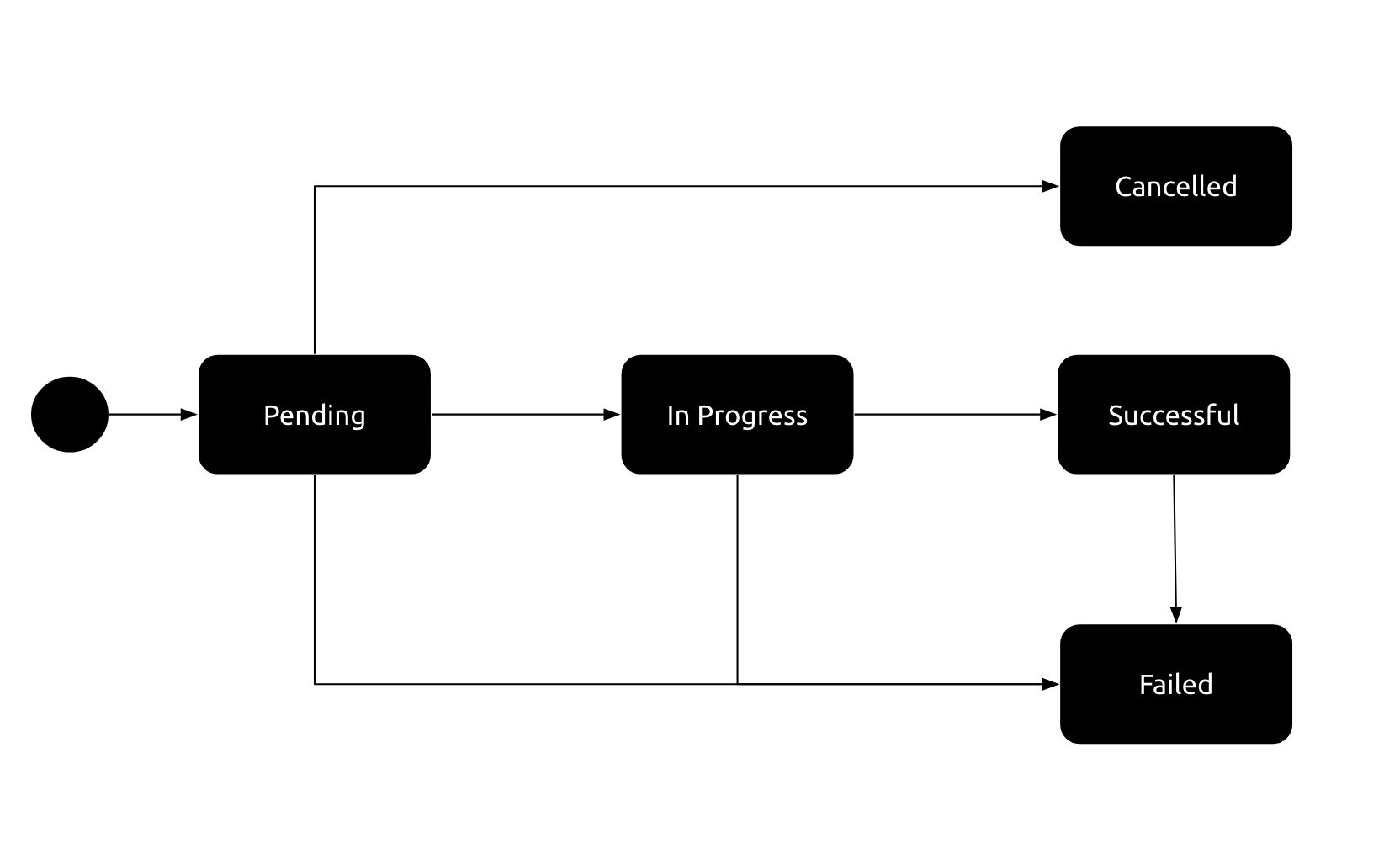
For more information, please visit our API Documentation
Updated 3 months ago